iOS (Native)
The Appnomix Commerce SDK is a library designed to help brands deepen their relationship with customers by placing the brand prominently on the users' mobile phones.
Minimum requirements
- iOS version 15+
- Xcode 15.4
Setup
Follow the instructions below to setup the Appnomix Commerce SDK in your existing iOS application.
For Automated Setup, refer to the instructions at this link. Otherwise, you can follow the manual steps later in this guide.
For any questions regarding the integration, please contact [email protected].
Safari Extension
-
Download & Copy the
Appnomix.Safari.Extension.xctemplate.zip
file from the assets of the latest release. (download link).-
To locate the Xcode folder, follow these steps:
- Open a new Finder window.
- Navigate to the
Go
menu at the top of the screen. - Select
Go to Folder...
- In the dialog box that appears, type
~/Library/Developer/Xcode
and press Enter.
- Make a new folder named
Templates
. - Copy the
Appnomix.Safari.Extension.xctemplate
to theTemplates
folder.
-
-
Go to your project and add New Target using Appnomix Safari Extension template (see screenshots below).
- The new target name should be
[Your Product] Extension
- Update the extension's name and description by editing the file located at
Resources/_locales/en/messages.json
. - Replace the required logos in the
Resources/images
directory, ensuring the file names remain unchanged.
- The new target name should be
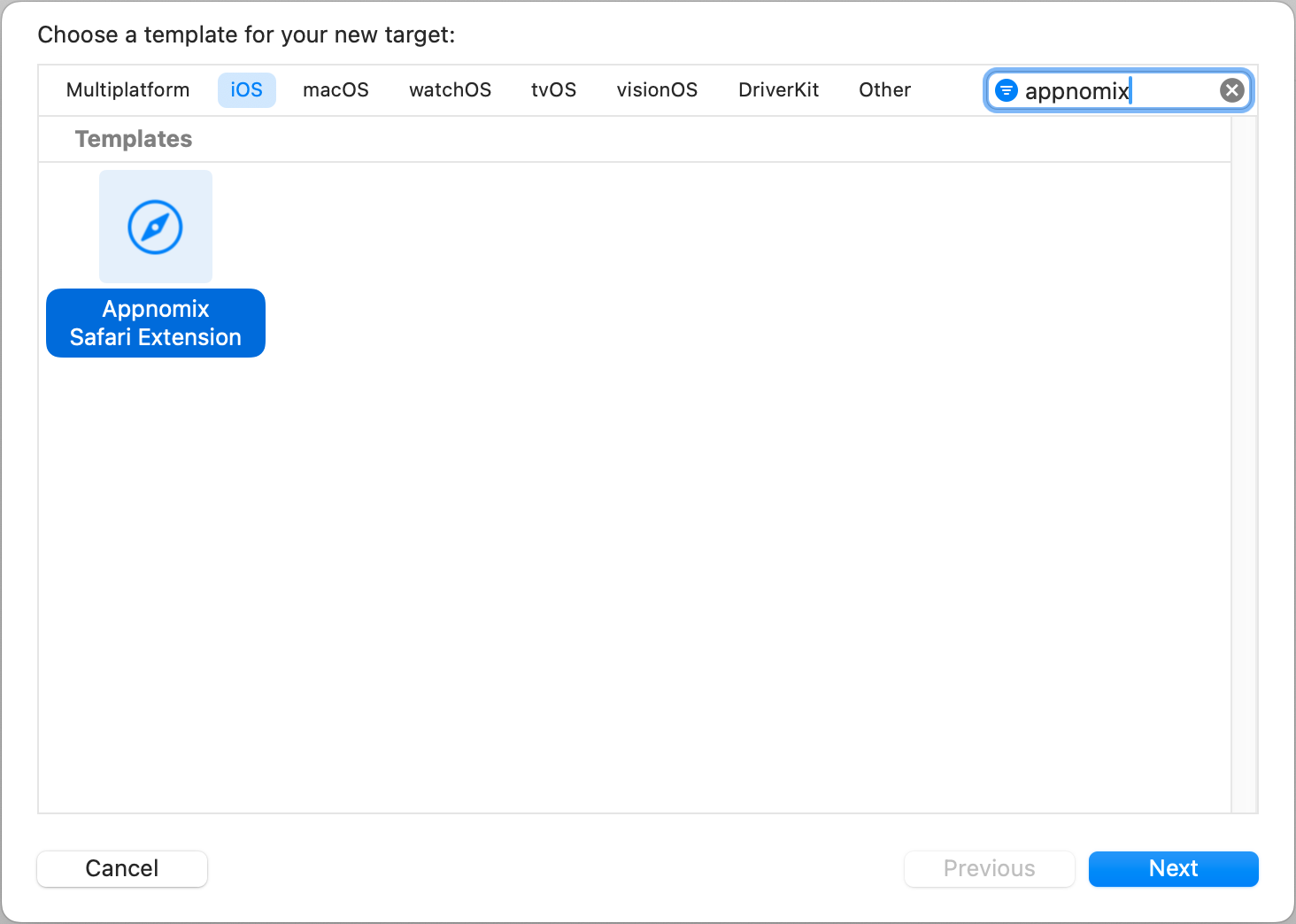
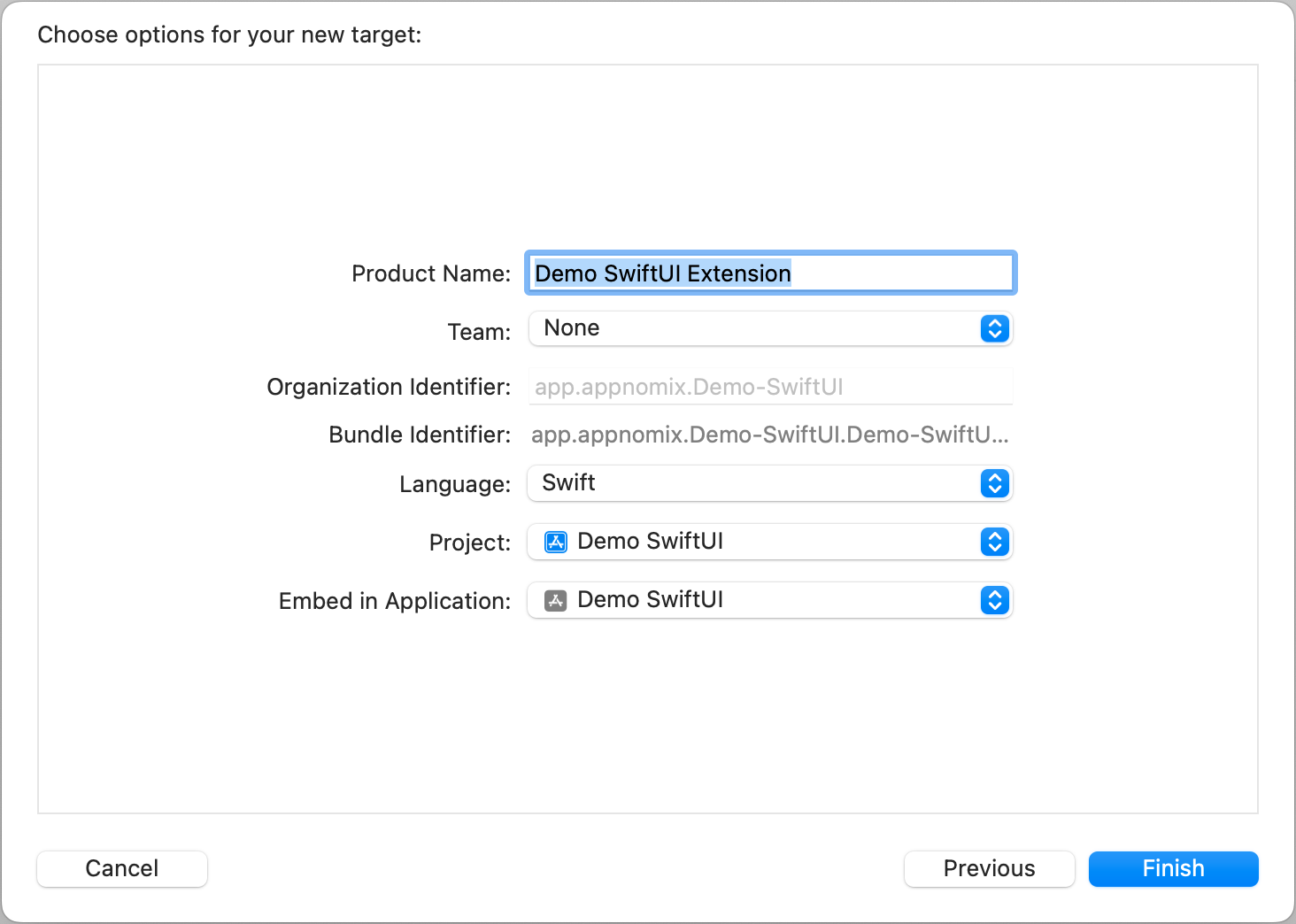
SDK integration
- Go to your project and add package dependency from GitHub.
- Use this source:
https://github.com/NomixGroup/ios_commerce_sdk_binary/
- Make sure you select Dependency Rule: Up to Next Major Version
- Use this source:
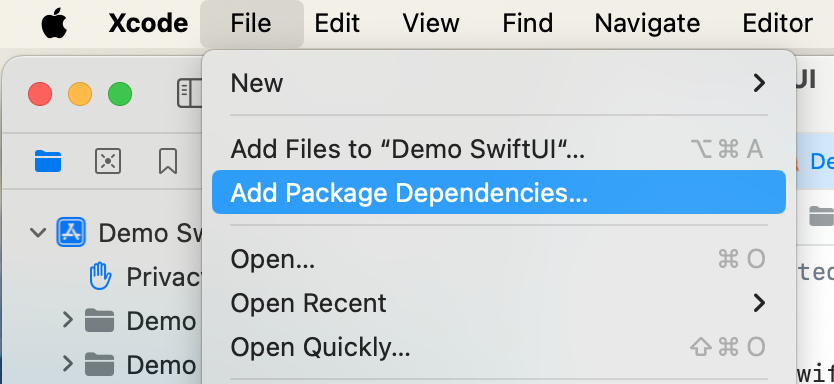
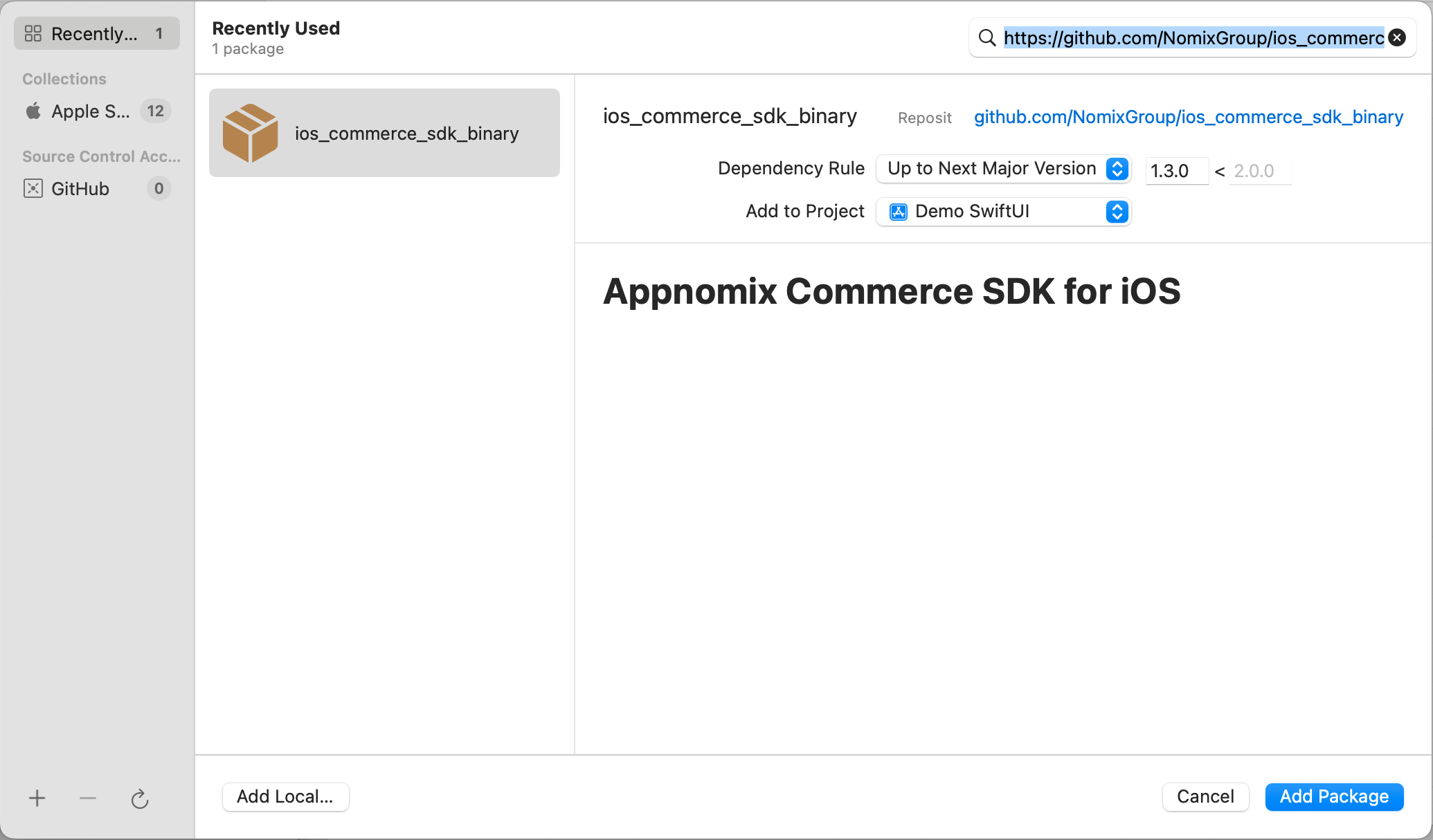
- Add AppnomixCommerce library to your main app target and the new created Extension target.
- Make sure you add the library for both targets: Your App and Safari Extension
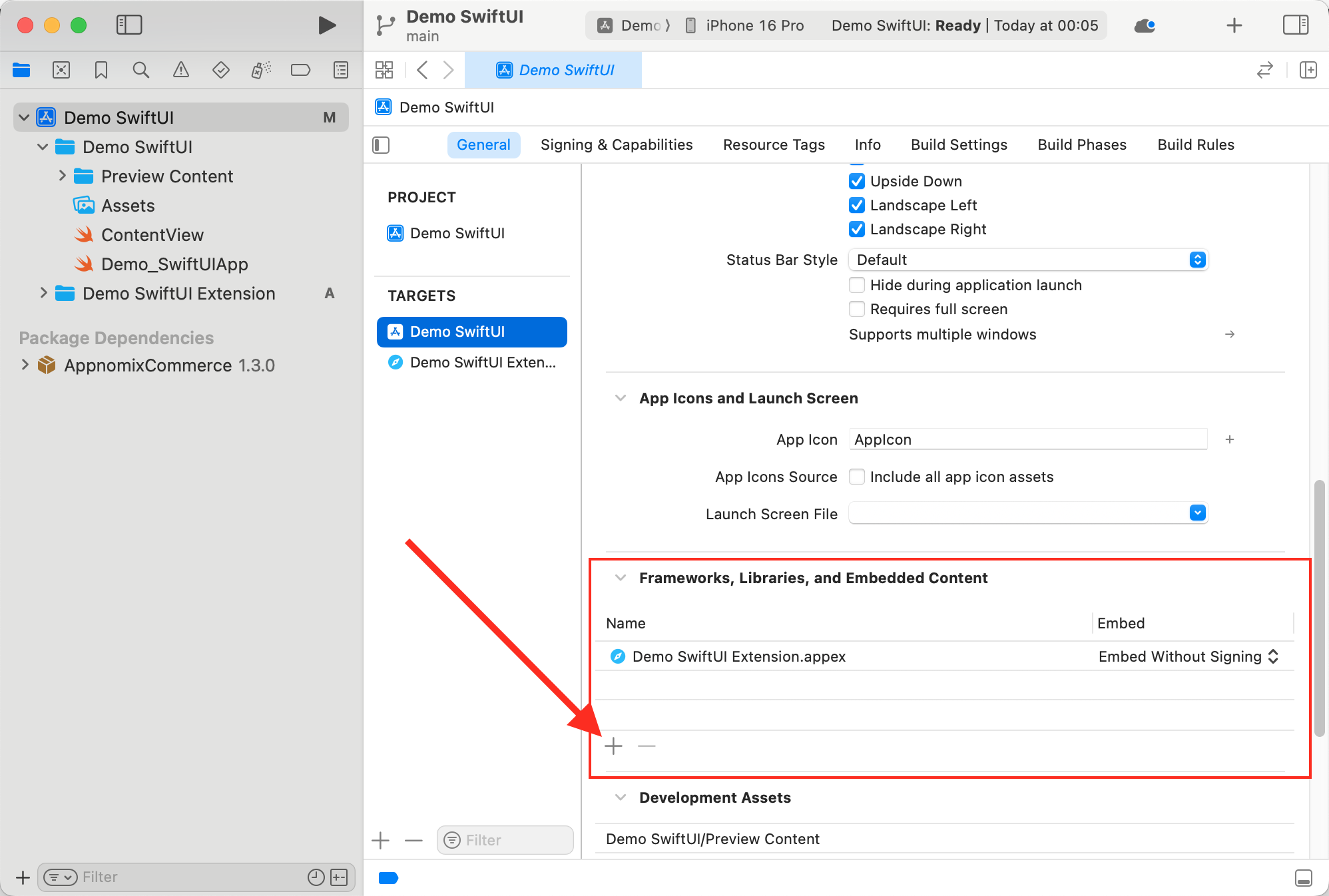
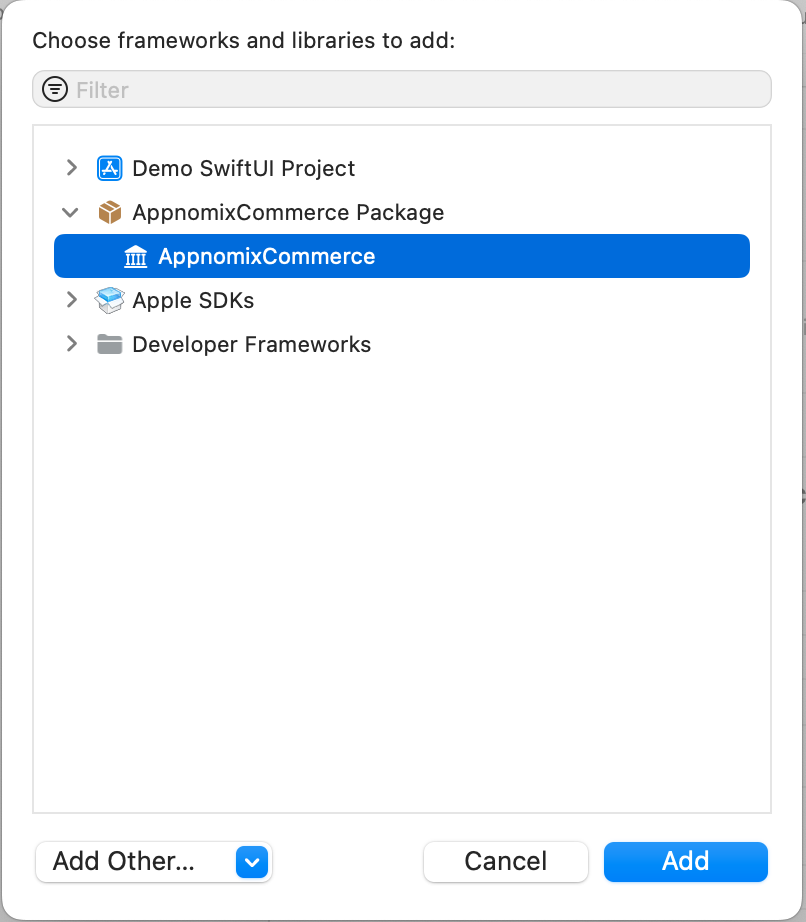
- Integrate AppnomixCommerceSDK - your code needs modifications in four sections:
- Import the AppnomixCommerce SDK.
- Ensure that the
AppDelegate
and theonboardingEvent
variable are properly defined. - Integrate the Appnomix Onboarding screen to boost the adoption rate of the extension.
- Incorporate the AppnomixCommerceSDK initializer into your
AppDelegate
and tailor your data accordingly:- Obtain
YOUR_CLIENT_ID
andYOUR_AUTH_TOKEN
by contacting [email protected]. appGroupName
(required) Set the App Group name to enable shared data between the app and the extension.- Important note: To ensure that your Safari extension operates effectively, you must activate the App Groups capability for both your app and the extension's targets. You can do this by following the steps outlined here: Configuring App Groups.
appURLScheme
(optional) Set the custom URL scheme of your app.- Learn how to define a custom URL scheme for your app by visiting: Defining a Custom URL Scheme for Your App.
requestLocation
(optional) Set this totrue
if you want the SDK to request access to the user's location information.- Add
NSLocationWhenInUseUsageDescription
to your Info.plist. For more information, visit: NSLocationWhenInUseUsageDescription.
- Add
requestTracking
(optional) Set this totrue
if you want the SDK to request the permission to use data for tracking the user's device. The following instruction is required if this parameter is set totrue
.- Add
NSUserTrackingUsageDescription
to your Info.plist. For more information, visit: NSUserTrackingUsageDescription.
- Add
- Obtain
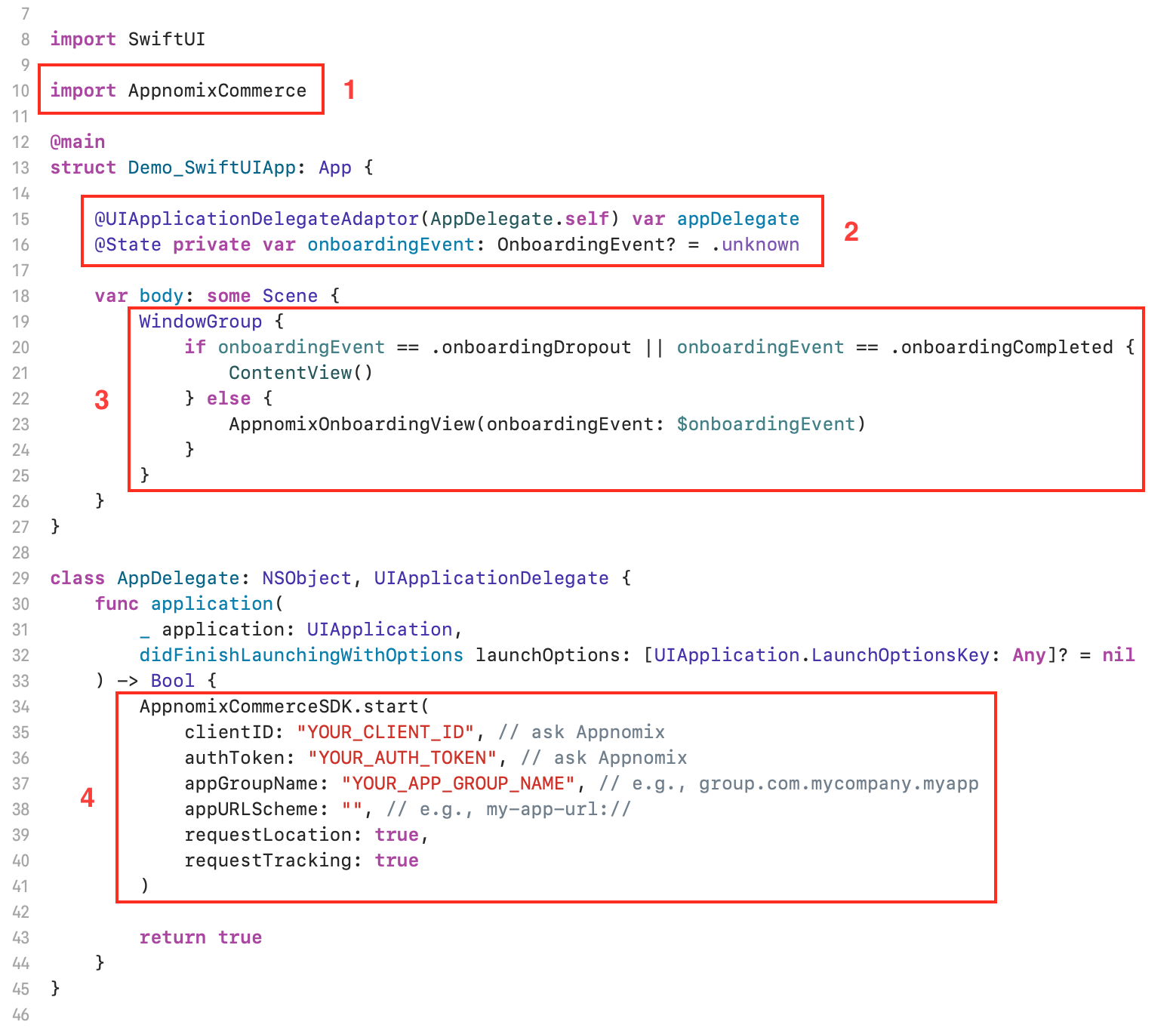
SDK Public Methods
AnalyticsFacade().trackOfferDisplay
- This method tracks when the Appnomix offer is displayed to the user. You must call this function when your screen displays CTA for accessing the offer. In the case of starting the app with the SDK onboarding, you must call this function after starting the SDK.AppnomixCommerceSDK.isExtensionInstalled()
- This method checks whether the browser extension is currently considered installed and active.- Returns
true
if the extension is enabled and the SDK has detected user activity in the browser within the past 14 days.
Returnsfalse
otherwise.
- Returns
Sample code
- You can use the code below, to copy and adapt to your application code.
import SwiftUI
import AppnomixCommerce
@main
struct Demo_SwiftUIApp: App {
@UIApplicationDelegateAdaptor(AppDelegate.self) var appDelegate
@State private var onboardingEvent: OnboardingEvent? = .unknown
var body: some Scene {
WindowGroup {
if AppnomixCommerceSDK.isExtensionInstalled() || onboardingEvent == .onboardingDropout || onboardingEvent == .onboardingCompleted {
ContentView()
} else {
AppnomixOnboardingView(onboardingEvent: $onboardingEvent)
}
}
}
}
class AppDelegate: NSObject, UIApplicationDelegate {
func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]? = nil
) -> Bool {
AppnomixCommerceSDK.start(
clientID: "YOUR_CLIENT_ID", // ask Appnomix
authToken: "YOUR_AUTH_TOKEN", // ask Appnomix
appGroupName: "YOUR_APP_GROUP_NAME", // e.g., group.com.mycompany.myapp
appURLScheme: "", // e.g., my-app-url://
requestLocation: true,
requestTracking: true
)
AnalyticsFacade().trackOfferDisplay(context: "app_start")
return true
}
}
- Set your App Group Name to SafariWebExtensionHandler.swift file:
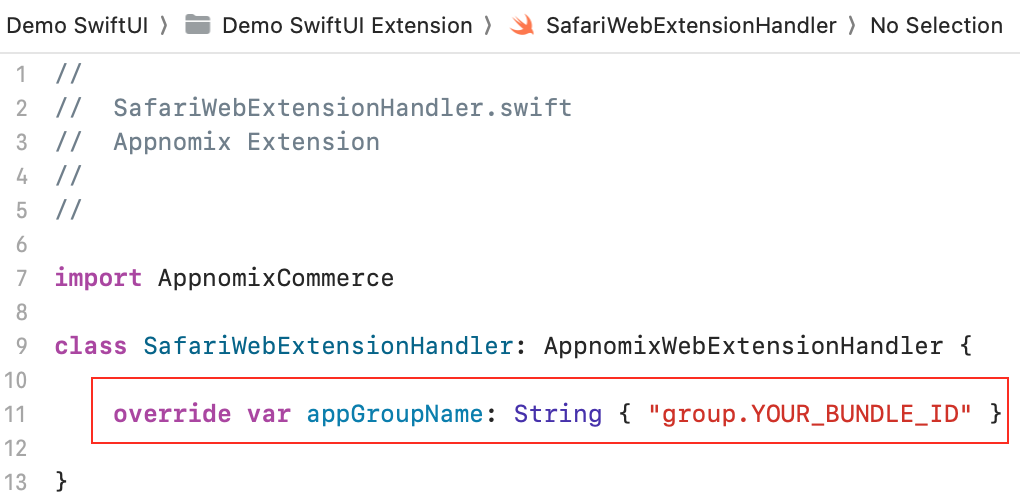
Analytics Events
The OnboardingEvent
(refer to the code snippet above) allows you to execute custom actions at each stage of the onboarding process and track a user's progress through the onboarding flow.
Below is a list of the available events:
OnboardingEvent.onboardingStarted
: Triggered when the onboarding screen is displayed.OnboardingEvent.onboardingDropout
: Triggered when the user cancels the onboarding process.OnboardingEvent.onboardingCompleted
: Triggered upon onboarding completion, indicating that the extension was installed.
API Declaration
Starting May 1, 2024, Apple mandates that apps utilizing APIs requiring justification must declare them in their app's privacy manifest. Here are the steps to include or update your privacy manifest with the APIs utilized by the Appnomix Extension.
- Add the
PrivacyInfo.xcprivacy
file to your Xcode project by following the illustrated steps below:
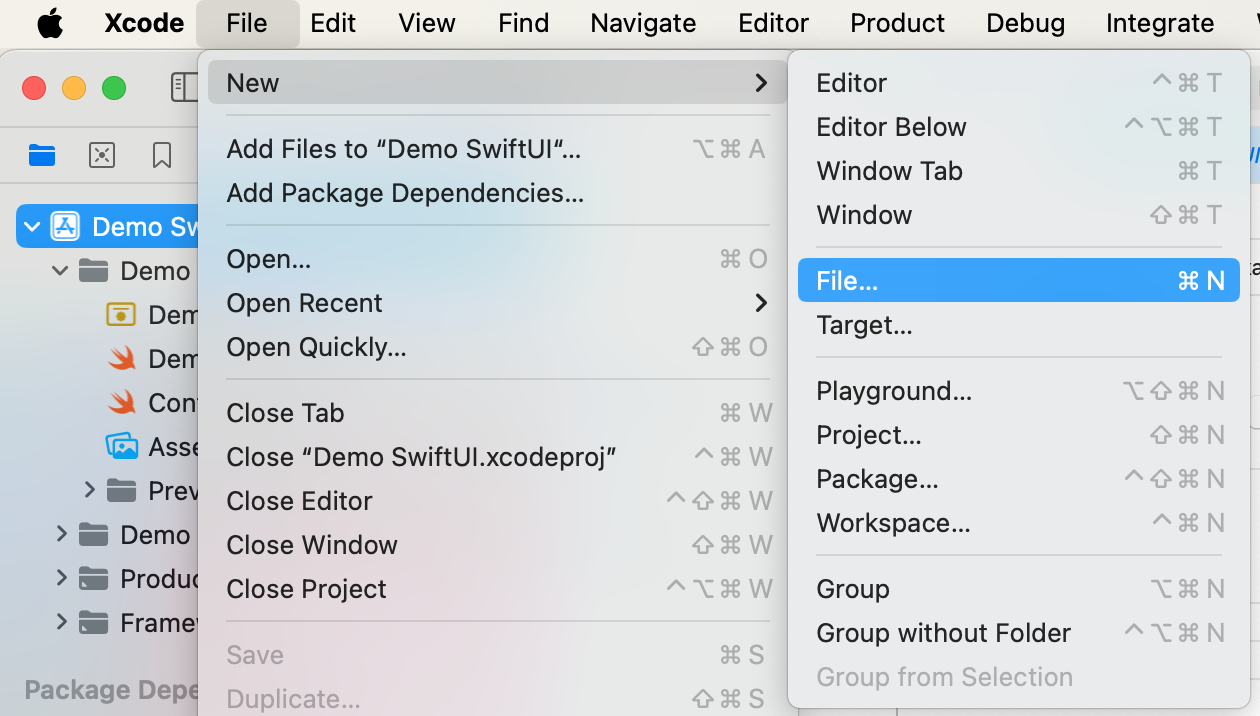
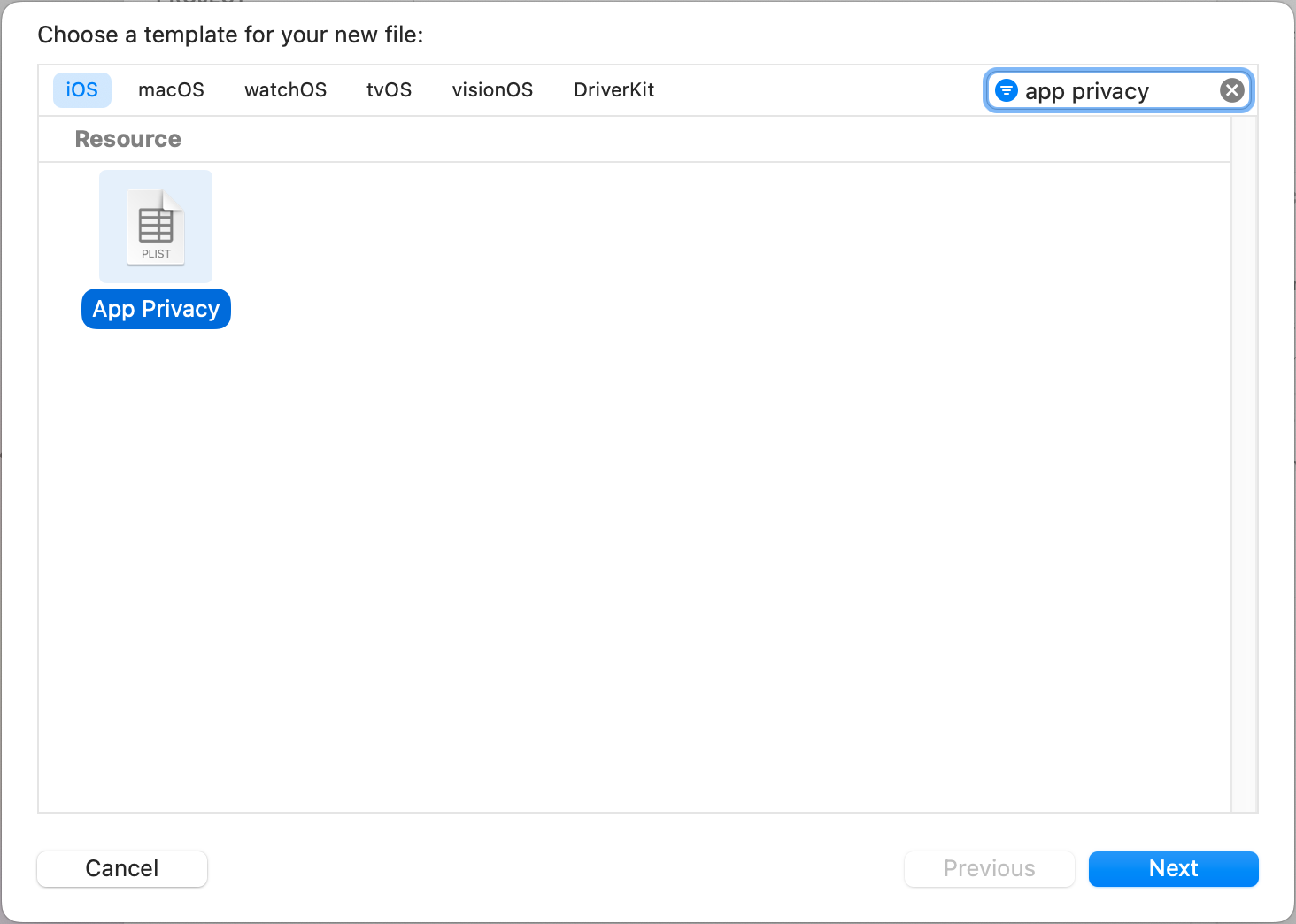
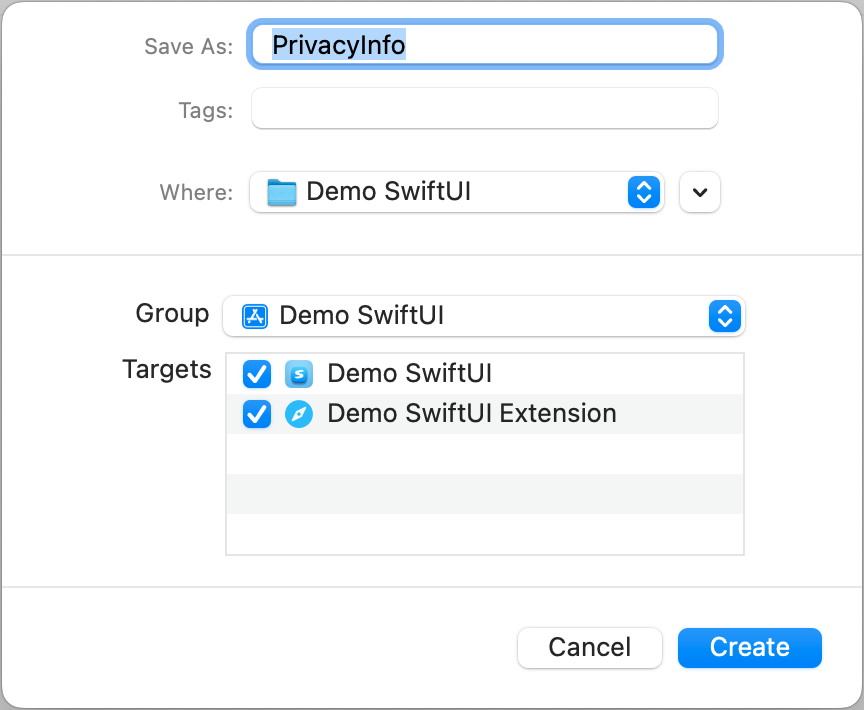
- Include the entries detailed below as API types and their corresponding reasons:
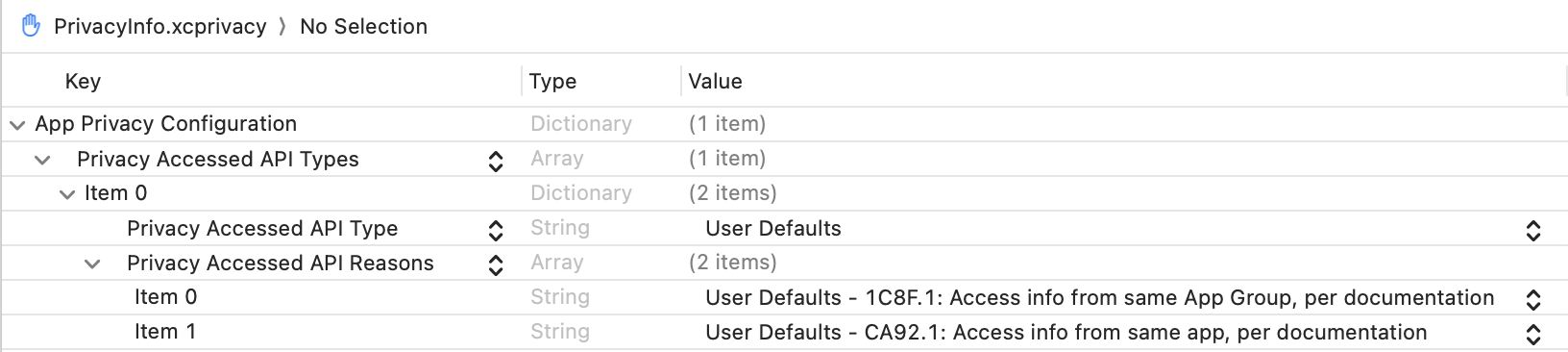
- Here is an example of how the
PrivacyInfo.xcprivacy
file should look:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>NSPrivacyAccessedAPITypes</key>
<array>
<dict>
<key>NSPrivacyAccessedAPIType</key>
<string>NSPrivacyAccessedAPICategoryUserDefaults</string>
<key>NSPrivacyAccessedAPITypeReasons</key>
<array>
<string>1C8F.1</string>
<string>CA92.1</string>
</array>
</dict>
</array>
</dict>
</plist>
How to Update the Safari Extension
- Download and extract the latest zip file provided in the Safari Extension.
- Open Finder to access the folder of your Safari Extension within your Xcode project (e.g.
[Your Product] Extension
) - Make sure you backup your customized files:
Resources/_locales/en/messages.json
.Resources/images
SafariWebExtensionHandler.swift
- Replace the contents of the
Appnomix Extension
with the folder downloaded in step 1. - Copy the backed-up files from step 3 back to their respective locations.
Automated Setup
The SetupXcode.sh script helps you integrate the Safari extension into your Xcode project and automatically configures all necessary settings. Just follow these simple steps:
- Download and unzip SetupXcode.sh.zip file into the same folder as your Xcode project.
- Place your logo image as
logo.png
in the same folder asSetupXcode.sh
. - Open
SetupXcode.sh
in a text editor and update theAPP_GROUPS_NAME
variable with your app group identifier. - Open Terminal, navigate to the folder, and run the script:
./SetupXcode.sh
Customize Onboarding screen
You can customize the colors on the onboarding screen by configuring and adding the AppnomixCustomizationPoints.json file to your project.
You can also see how the above can be integrated with our Appnomix SwiftUI Sample.
Wishing you a smooth integration!
Updated 13 days ago