Unity Integration
Instructions to integrate the Appnomix Commerce SDK with your Unity project for iOS and Android.
Minimum requirements
- iOS
- iOS version 15
- Xcode 15.4
- Android
- minSDK version 5.0 (API 21)
- Android compileSDK version 15 (API 35)
- Java 17
- Android Gradle Plugin 8.0.2
- Unity 2022.3
- A valid installation of Unity and Xcode
Setup
Follow the instructions below to setup the Appnomix Commerce SDK in your existing Unity project.
For any questions regarding the integration, please contact [email protected].
Import the Unity package
- Download the latest AppnomixCommerceSDK package for Unity from here.
- In Unity, go to Assets > Import Package > Custom Package…
- Select the Unity package you previously downloaded (unity-<version>.unitypackage).
- In the Import Unity Package dialog box, click on the Import button.
Note for iOS build: The Unity package includes a script SetupXcode.sh that automates the Xcode setup process, so you don't need to take any additional steps for that.
Android (only)
If not already available in your project, install External Dependency Manager for Unity and ensure 'Patch mainTemplate.gradle' is checked, via Assets > External Dependency Manager > Android Resolver > Settings.
The Plugin doesn't enforce the Java version and Android Gradle Plugin version so you'll need to set these yourself, in the gradle templates, in case the build fails.
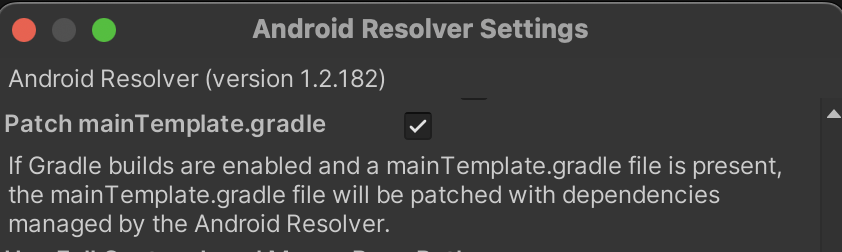
C# Binding
- Create a C# binding to initialize the SDK and launch the Appnomix onboarding screen. Below is an example of a
MonoBehavior
that can load in a Unity scene or can just invoke it directly.
You can callLaunchOnboarding
either from a scene or another C# script.
using UnityEngine;
using AppnomixCommerceSDK.Scripts;
public class StartSDK : MonoBehaviour
{
void Start()
{
AppnomixCommerceSDKWrapper sdk = new AppnomixCommerceSDKWrapper(
"_YOUR_APPNOMIX_CLIENT_ID_", // clientID
"_YOUR_APPNOMIX_AUTH_TOKEN_" // authToken
"_YOUR_APP_GROUP_iOS_", // iOSAppGroupName (e.g. group.app.appnomix.demo-unity)
"_YOUR_URL_SCHEME_iOS_", // iOSAppURLScheme (e.g. savers-league-coupons://)
true, // requestLocation
true // requestTracking
);
if (!sdk.IsOnboardingDone())
{
Debug.Log("Appnomix onboarding is starting.");
sdk.LaunchOnboarding(HandleAnalyticsEvent);
sdk.TrackOfferDisplay("app_start");
} else {
Debug.LogError("Appnomix onboarding is completed.");
}
}
[AOT.MonoPInvokeCallback(typeof(AnalyticsEventCallback))]
public static void HandleAnalyticsEvent(long eventValue)
{
Debug.Log($"Analytics event received: {eventValue}");
var analyticsEvent = (AnalyticsEvent)eventValue;
switch (analyticsEvent)
{
case AnalyticsEvent.OnboardingStarted:
// The event triggered when onboarding begins by displaying the screen
break;
case AnalyticsEvent.OnboardingDropout:
// The event triggered when the user cancels the onboarding process
break;
case AnalyticsEvent.OnboardingCompleted:
// The event triggered upon onboarding completion,
// indicating that the extension was installed and/or permissions were granted
break;
default:
// Handle unknown event or default case
break;
}
}
}
AppnomixCommerceSDK Parameters
clientID
andauthToken
- Obtain
YOUR\_CLIENT\_ID
andYOUR\_AUTH\_TOKEN
by contacting [email protected].
- Obtain
iOSAppGroupName
[mandatory][iOS only] - This value should look likegroup.YOUR\_BUNDLE\_ID
- Important note: To ensure that your Safari extension operates effectively, you must activate the App Groups capability for both your app and the extension's targets. You can do this by following the steps outlined here: Configuring App Groups.
iOSAppURLScheme
[optional][iOS only] - Set the custom URL scheme of your app or set an empty string.- Learn how to define a custom URL scheme for your app by visiting: Defining a Custom URL Scheme for Your App.
requestLocation
Set this totrue
if you want the SDK to request access to the user's location information.requestTracking
Set this totrue
if you want the SDK to request the permission to use data for tracking the user's device.- The .cs file needs to be loaded in Unity.
- The build process might not succeed, iterate until the issues are solved. For example: the .cs file might not compile because the class name does not coincide with the file name (i.e. class StartSDK with StartSDK.cs)
SDK Public Methods
IsOnboardingDone
- This method returnsTrue
if the onboarding process is complete. Onboarding is considered complete once the Safari extension is activated on iOS or the Accessibility permission is granted on Android.TrackOfferDisplay
- This method tracks when the Appnomix offer is displayed to the user. You must call this function when your screen displays CTA for accessing the offer. In the case of starting the app with the SDK onboarding, you must call this function after starting the SDK.
Analytics Events
The HandleAnalyticsEvent
method (refer to the code snippet above) allows you to execute custom actions during each stage of the onboarding process.
Below is a list of the available events:
AnalyticsEvent.OnboardingStarted
: Triggered when the onboarding screen is displayed.AnalyticsEvent.OnboardingDropout
: Triggered when the user cancels the onboarding process.AnalyticsEvent.OnboardingCompleted
: Triggered upon onboarding completion, indicating that the extension was installed and/or permissions were granted.
Update Safari Extension icon for iOS
- Replace the logo.png from [Assets/AppnomixCommerceSDK/Resources] with your logo.
- The recommended size is 512x512 pixels.
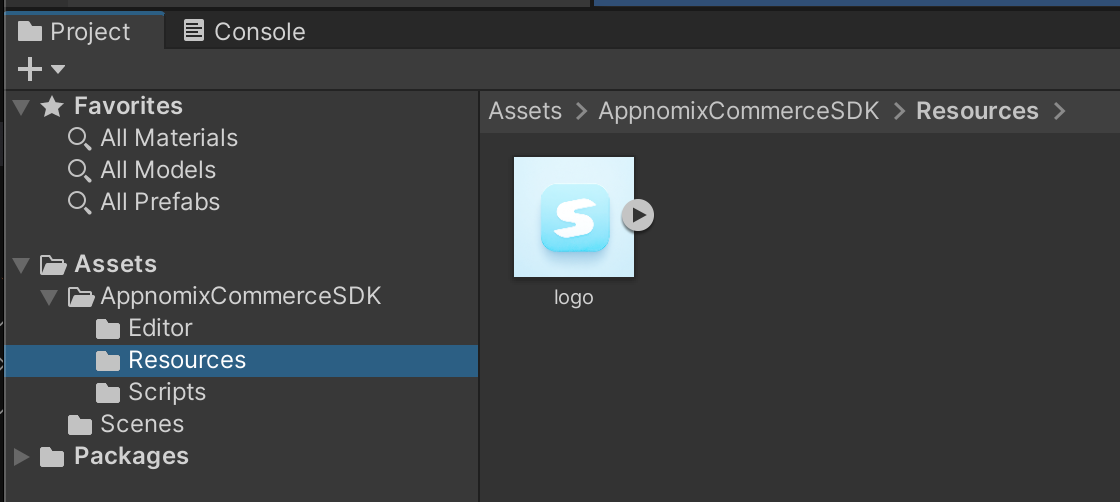
Customize Onboarding screen
- To customize the colors on the onboarding screen, download, configure and add the AppnomixCustomizationPoints.json file to [Assets/AppnomixCommerceSDK/Resources] in your Unity project.
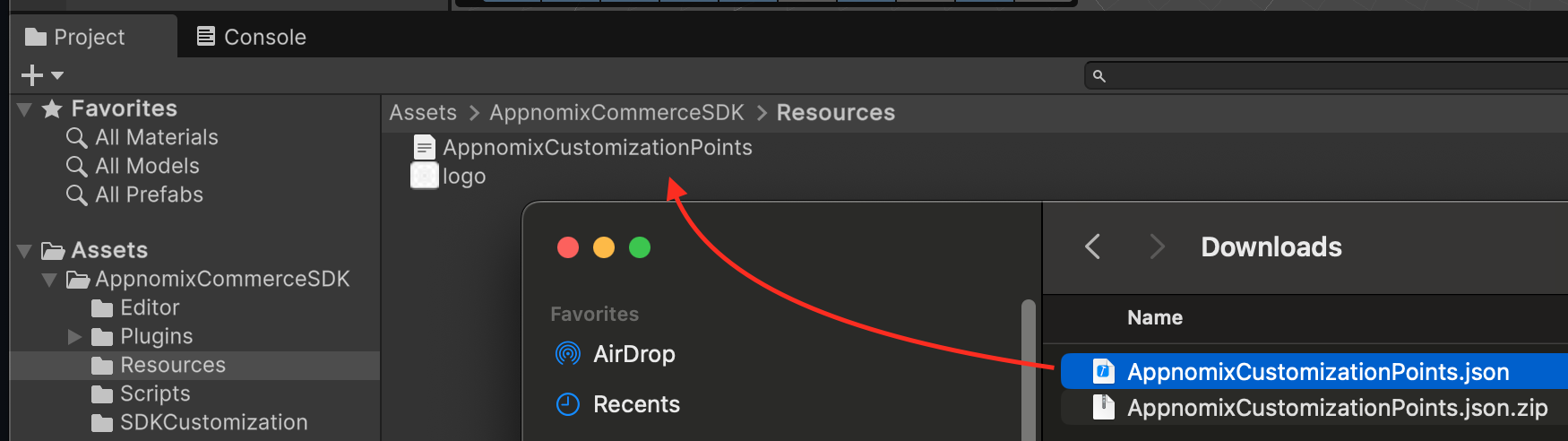
Wishing you a smooth integration!
Updated about 1 month ago